This Tutorial will allow you to create your very own WYSIWYG (What You See Is What You Get) Web Editor using the Web Browser Control and the mshtml Component with Open / Save HTML Support.
Printer Friendly Download Tutorial (468KB) Download Source Code (14.9KB)
Step 1
Start Microsoft Visual Basic 2008 Express Edition, then select File then New Project... Choose Windows Forms Application from the New Project Window, enter a name for the Project and then click OK, see below:
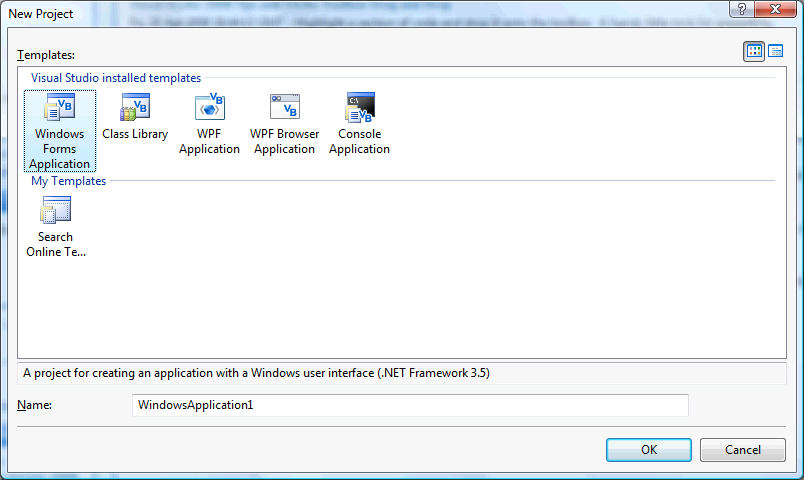
Step 2
A Blank Form named Form1 should then appear, see below:
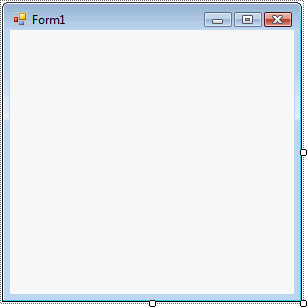
Step 3
With Form1 selected goto the Properties box and change the Name from Form1 to frmMain
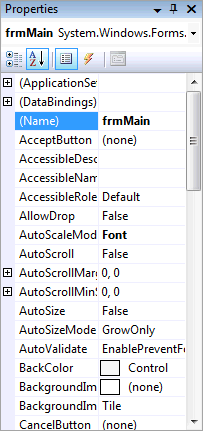
Step 4
Then select Project, then select "Add Reference...", make sure that Microsoft.mshtml component is selected, if not use the Browse tab and locate the "mshtml.tlb" file on your computer (use the Windows Search feature). If it is available it will appear in the list as below:
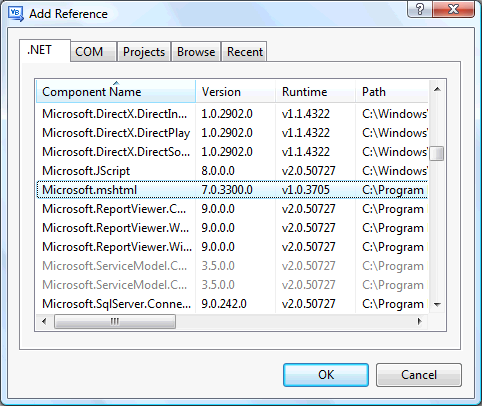
Step 5
Add the Reference to mshtml by clicking on Ok, from the Common Controls tab on the Toolbox select the WebBrowser component:
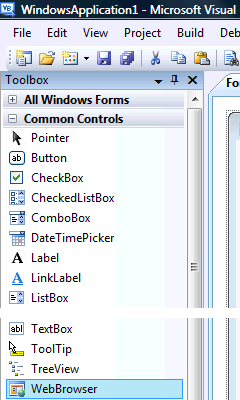
Step 6
Draw a WebBrowser on the Form, see below:
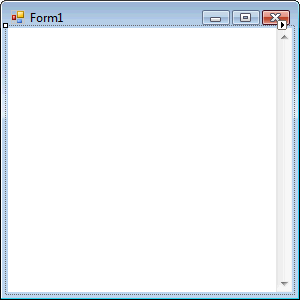
Step 7
Select or click on the WebBrowser Component, then goto the Properties box and change the name to "webEditor", then set the Dock property to "Fill" if not already set, all without quotes, see below:
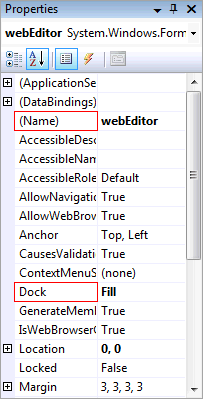
Step 8
Then from the Menus & Toolbars tab on the Toolbox select the MenuStrip Control, see Below:
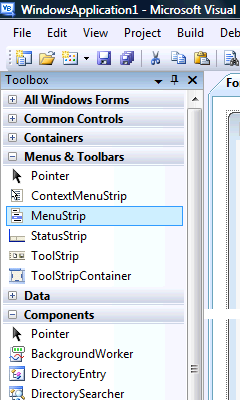
Step 9
Either double-click on the MenuStrip Control entry in the Menu & Toolbars tab on the Toolbox or keep the MenuStrip Component Clicked and then move it over the Form then let go. Change the Name of the MenuStrip in the properties to mnuWebEditor The Form will then look as below:
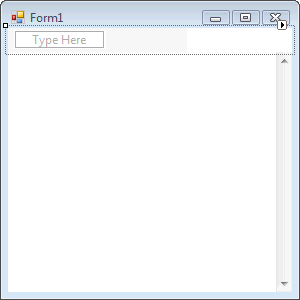
Step 10
Click or select where the MenuStrip says "Type Here", an editable Textbox will appear type in "File" without the quotes in this Box, then in the Box below that type in "New" then after that, "Open...", then below that "Save...", then "-" this is a minus or hyphen key and will show as a Separator and finally "Exit" all without quotes. The MenuStrip should look as below:
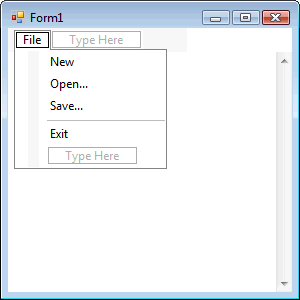
Step 11
Click or select where the MenuStrip says "Type Here" next to "File", and in this type in "Edit" without the quotes in this Box, then in the Box below that type "Cut", then below that "Copy", then "Paste" and "Delete", type in "-" which is minus or the hyphen key for a Seperator, then below that "Select All" and finally "Time/Date". The MenuStrip should look as below:
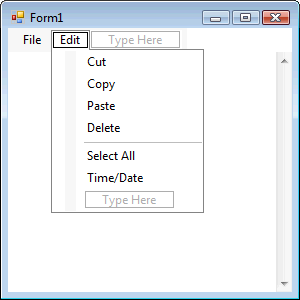
Step 12
Click or select next to "Edit" where the MenuStrip says "Type Here", and type in "Insert" without the quotes in this Box, then in the Box below that type in "Title...", below that "Link...", then "Image..." and finally "Divider". The MenuStrip should look as below:
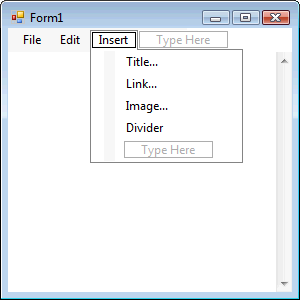
Step 13
Click or select next to "Insert" where the MenuStrip says "Type Here", and type in "Format" without the quotes in this Box, then in the Box below that type in "Font...", then below that "Colour..." then "Background...", then "-" which is minus or the hyphen key for a Separator, then below that type "Align Left", then "Align Centre" then finally "Align Right", see below:
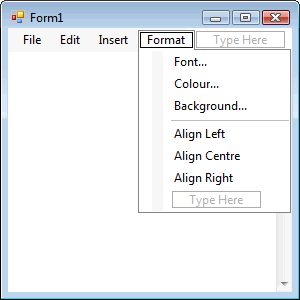
Step 14
Right Click on the Form or the entry of the Form in Solution Explorer and choose the "View Code" option then above the "Public Class frmMain" type the following:
Imports mshtml
Also while still in the Code View, below the "Public Class frmMain" part type the following Declaration and Subroutine:
Public doc As mshtml.IHTMLDocument2 Public Sub Command(ByRef Name As String, _ Optional ByVal Value As Object = Nothing) doc.execCommand(Name, False, Value) End Sub
See Below:
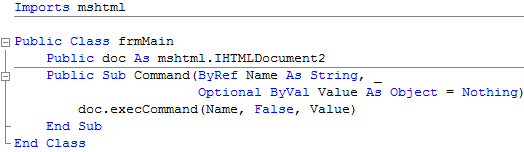
Step 15
While still in the Code View for frmMain, enter the following Function and Subroutine above the "End Class" and below the "End Sub" of "Public Sub Command()":
Public Function SelectedItem(ByVal Command As String) As Object Dim Selection As IHTMLSelectionObject = doc.selection ' Get Selection If Not Selection Is Nothing Then Dim Range As IHTMLTxtRange = Selection.createRange ' Get the Range If Range.queryCommandSupported(Command) And _ Range.queryCommandEnabled(Command) Then Return Range.queryCommandValue(Command) End If End If Return Nothing End Function Public Sub SelectedText(ByVal Value As String) Dim Selection As IHTMLSelectionObject = doc.selection ' Get Selection If Not Selection Is Nothing Then Dim Range As IHTMLTxtRange = Selection.createRange ' Get the Range If Not Range Is Nothing Then Range.pasteHTML(Value) End If End If End Sub
See Below:
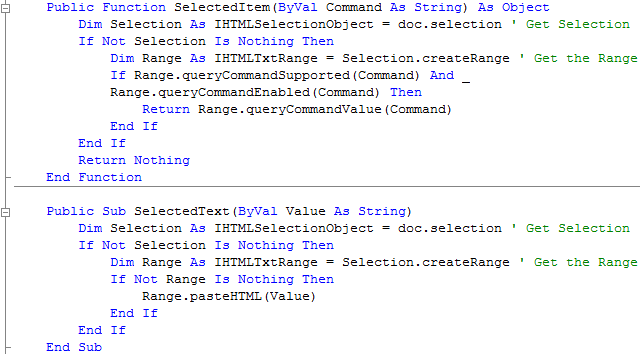
Step 16
Again while still in the Code View for frmMain, below the "End Sub" of "Public Sub SelectedText()", enter the following Functions
Private Function FontSizeToHTML(ByVal Size As Integer) As Integer If Size < 10 Then Return 1 ElseIf Size < 12 Then Return 2 ElseIf Size < 14 Then Return 3 ElseIf Size < 18 Then Return 4 ElseIf Size < 24 Then Return 5 ElseIf Size < 36 Then Return 6 Else Return 7 End If End Function Private Function FontSizeFromHTML(ByRef Size As Integer) As Single Select Case Size Case 1 Return 8.0F Case 2 Return 10.0F Case 3 Return 12.0F Case 4 Return 14.0F Case 5 Return 18.0F Case 6 Return 24.0F Case 7 Return 36.0F Case Else Return 12.0F End Select End Function
See Below:
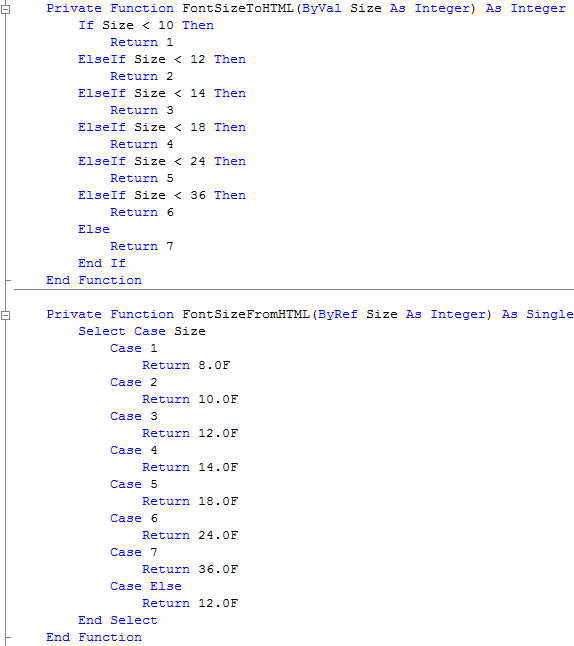
Step 17
Still in the Code View for frmMain, below the "End Function" of "Private Function FontSizeFromHTML()", enter the following Function and Subroutine
Public Function GetFormatFont() As Font Try Dim FontName As String = SelectedItem("FontName") Dim FontSize As String = FontSizeFromHTML(SelectedItem("FontSize")) Dim IsBold As Boolean = SelectedItem("Bold") Dim IsItalic As Boolean = SelectedItem("Italic") Dim TheFontStyle As Integer = 0 If IsBold Then TheFontStyle = FontStyle.Bold If IsItalic Then TheFontStyle = FontStyle.Italic If IsBold And IsItalic Then TheFontStyle = FontStyle.Bold + FontStyle.Italic If Not (IsBold And IsItalic) Then TheFontStyle = FontStyle.Regular Return New Font(FontName, FontSize, TheFontStyle, GraphicsUnit.Point) Catch ex As Exception Return Nothing End Try End Function Public Sub FormatFont(ByRef Font As Font) Command("FontName", Font.Name) Command("FontSize", FontSizeToHTML(Font.Size)) If Font.Bold Then Command("Bold") End If If Font.Italic Then Command("Italic") End If End Sub
See Below:
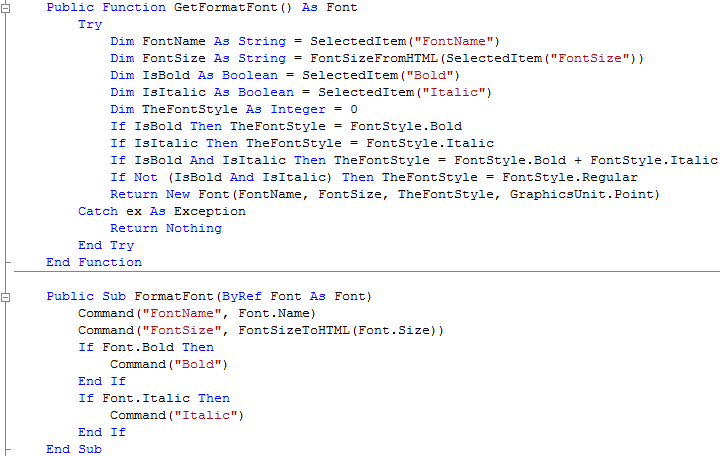
Step 18
Finally while still in the Code View for frmMain, below the "End Sub" of "Public Sub FormatFont()", enter the following Subroutines
Public Sub FormatColour(ByRef Colour As Color) Dim strColour As String If Not Colour.Equals(Color.Empty) Then strColour = ColorTranslator.ToHtml(Colour) Else strColour = Nothing End If Command("ForeColor", strColour) End Sub Public Sub FormatBackground(ByRef Colour As Color) Dim strColour As String If Not Colour.Equals(Color.Empty) Then strColour = ColorTranslator.ToHtml(Colour) Else strColour = String.Empty End If doc.bgColor = strColour End Sub
See Below:
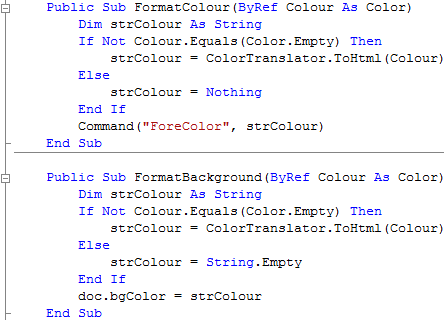
Step 19
Return to the Design view by selecting the [Design] tab or Right Click on the "View Designer" option in Solution Explorer for frmMain. Click on "File" on the MenuStrip, then Double Click on the Menu Item Labeled "New" (NewToolStripMenuItem) and type the following in the NewToolStripMenuItem_Click() Sub:
Dim Response As MsgBoxResult Response = MsgBox("Are you sure you want to start a New Document?", _ MsgBoxStyle.Question + MsgBoxStyle.YesNo, _ "Web Editor") If Response = MsgBoxResult.Yes Then doc.clear() webEditor.Navigate("about:blank") ' New Document Me.Text = "Web Editor - Untitled" End If
See Below:
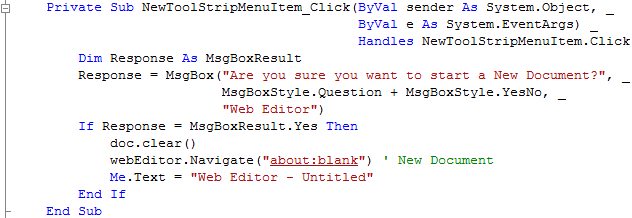
Step 20
Click on the [Design] Tab to view the form again, then Click on "File" on the MenuStrip, then Double Click on the Menu Item Labeled "Open..." (OpenToolStripMenuItem) and type the following in the OpenToolStripMenuItem_Click() Sub:
Dim Open As New OpenFileDialog() Open.Filter = "HTML Files (*.html,*.htm)|*.html;*.htm|All files (*.*)|*.*" Open.CheckFileExists = True If Open.ShowDialog(Me) = Windows.Forms.DialogResult.OK Then Try webEditor.Navigate(Open.FileName) Me.Text = "Web Editor - " & Open.FileName Catch ex As Exception ' Do nothing on Exception End Try End If
See Below:
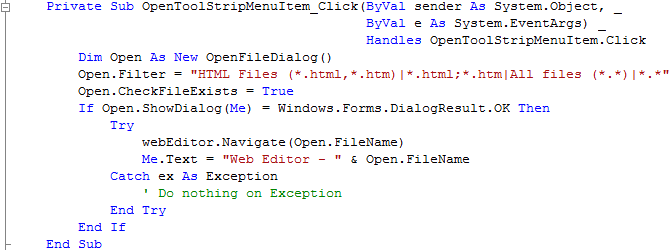
Step 21
Click on the [Design] Tab to view the form again, then Click on "File" on the MenuStrip, then Double Click on the Menu Item Labeled "Save..." (SaveToolStripMenuItem) and type the following in the SaveToolStripMenuItem_Click() Sub
Dim Save As New SaveFileDialog() Dim myStreamWriter As System.IO.StreamWriter Save.Filter = "HTML Files (*.html)|*.html|All files (*.*)|*.*" Save.CheckPathExists = True If Save.ShowDialog(Me) = Windows.Forms.DialogResult.OK Then Try myStreamWriter = System.IO.File.CreateText(Save.FileName) myStreamWriter.Write(webEditor.DocumentText) myStreamWriter.Flush() Me.Text = "Web Editor - " & Save.FileName Catch ex As Exception ' Do nothing on Exception End Try End If
See Below:
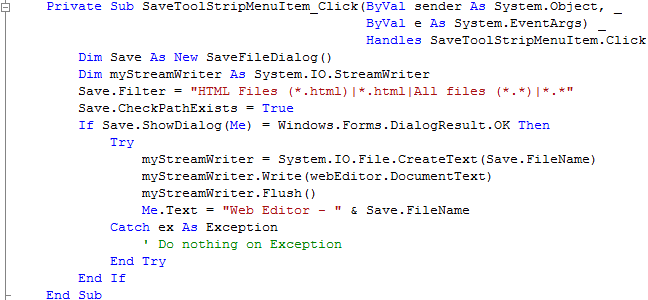
Step 22
Click on the [Design] Tab to view the form again, then Click on "File" on the MenuStrip, then Double Click on the Menu Item Labeled "Exit" (ExitToolStripMenuItem) and type the following in the ExitToolStripMenuItem_Click() Sub
Dim Response As MsgBoxResult Response = MsgBox("Are you sure you want to Exit Web Editor?", _ MsgBoxStyle.Question + MsgBoxStyle.YesNo, _ "Web Editor") If Response = MsgBoxResult.Yes Then End End If
See Below:
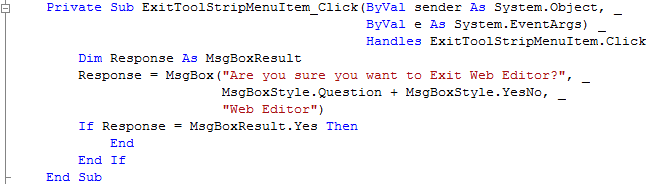
Step 23
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip, then Double Click on the Menu Item Labeled "Cut" (CutToolStripMenuItem) and type the following in the CutToolStripMenuItem_Click() Sub:
Command("Cut")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Edit", then Double Click on the Menu Item Labeled "Copy" (CopyToolStripMenuItem) and type the following in the CopyToolStripMenuItem_Click() Sub:
Command("Copy")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Edit", then Double Click on the Menu Item Labeled "Paste" (PasteToolStripMenuItem) and type the following in the PasteToolStripMenuItem_Click() Sub:
Command("Paste")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Edit", then Double Click on the Menu Item Labeled "Delete" (DeleteToolStripMenuItem) and type the following in the DeleteToolStripMenuItem_Click() Sub:
Command("Delete")
See Below:
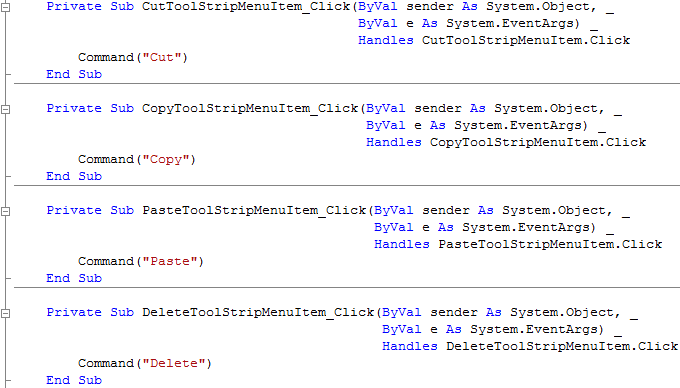
Step 24
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip, then Double Click on the Menu Item Labeled "Select All" (SelectAllToolStripMenuItem) and type the following in the SelectAllToolStripMenuItem_Click() Sub:
Command("SelectAll")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Edit", then Double Click on the Menu Item Labeled "Time/Date" (TimeDateToolStripMenuItem) and type the following in the TimeDateToolStripMenuItem_Click() Sub:
SelectedText(Format(Now, "HH:mm dd/MM/yyyy"))
See Below:
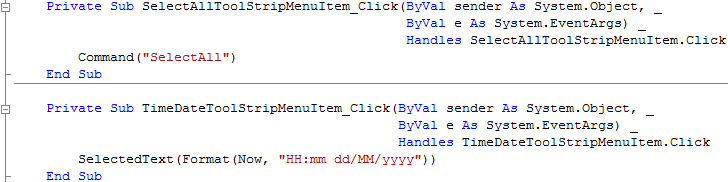
Step 25
Click on the [Design] Tab to view the form again, then Click on "Insert" on the MenuStrip, then Double Click on the Menu Item Labeled "Title..." (TitleToolStripMenuItem) and type the following in the TitleToolStripMenuItem_Click() Sub
Dim Response As String = "My Website" If webEditor.DocumentTitle <> "" Then Response = webEditor.DocumentTitle End If Response = InputBox("Title", "Web Editor", Response) If Response <> "" Then doc.title = Response End If
See Below:
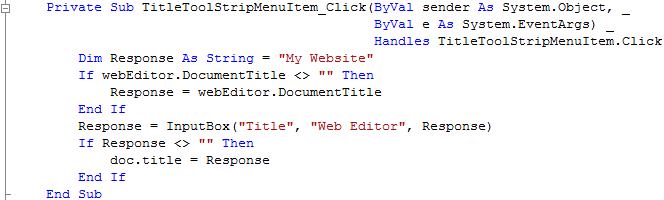
Step 26
Click on the [Design] Tab to view the form again, then Click on "Insert" on the MenuStrip, then Double Click on the Menu Item Labeled "Link..." (LinkToolStripMenuItem) and type the following in the LinkToolStripMenuItem_Click() Sub
Dim Response As String = "http://www.cespage.com/vb" Response = InputBox("Web Address", "Web Editor", Response) If Response <> "" Then Command("CreateLink", Response) End If
See Below:
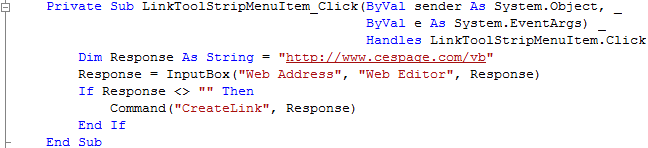
Step 27
Click on the [Design] Tab to view the form again, then Click on "Insert" on the MenuStrip, then Double Click on the Menu Item Labeled "Image..." (ImageToolStripMenuItem) and type the following in the ImageToolStripMenuItem_Click() Sub
Dim Response As String = "http://www.cespage.com/vb/images/vb08tut13.png" Response = InputBox("Image URL", "Web Editor", Response) If Response <> "" Then Command("InsertImage", Response) End If
See Below:
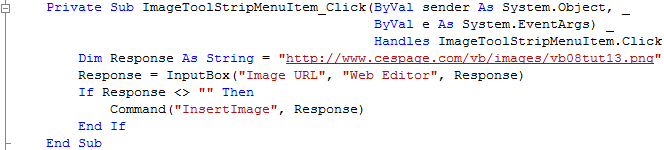
Step 28
Click on the [Design] Tab to view the form again, then Click on "Insert" on the MenuStrip, then Double Click on the Menu Item Labeled "Divider" (DividerToolStripMenuItem) and type the following in the DividerToolStripMenuItem_Click() Sub
Command("InsertHorizontalRule")
See Below:

Step 29
Click on the [Design] Tab to view the form again, then Click on "Format" on the MenuStrip, then Double Click on the Menu Item Labeled "Font..." (FontToolStripMenuItem) and type the following in the FontToolStripMenuItem_Click() Sub
Dim Font As New FontDialog() Font.MaxSize = 36 Font.ShowEffects = False Font.AllowScriptChange = False Font.Font = GetFormatFont() Font.ShowDialog(Me) Try FormatFont(Font.Font) Catch ex As Exception ' Do nothing on Exception End Try
See Below:
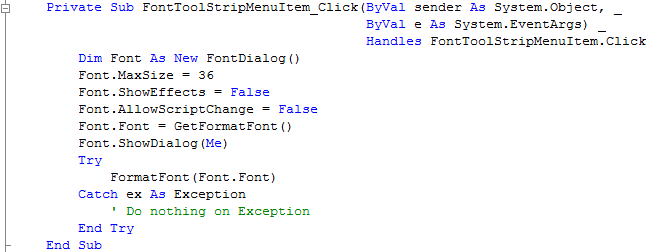
Step 30
Click on the [Design] Tab to view the form again, then Click on "Format" on the MenuStrip, then Double Click on the Menu Item Labeled "Colour..." (ColourToolStripMenuItem) and type the following in the ColourToolStripMenuItem_Click() Sub
Dim Colour As New ColorDialog() Colour.Color = ColorTranslator.FromHtml(doc.fgColor) Colour.ShowDialog(Me) Try FormatColour(Colour.Color) Catch ex As Exception ' Do nothing on Exception End Try
See Below:
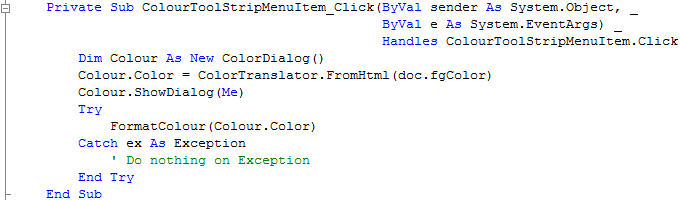
Step 31
Click on the [Design] Tab to view the form again, then Click on "Format" on the MenuStrip, then Double Click on the Menu Item Labeled "Background..." (BackgroundToolStripMenuItem) and type the following in the BackgroundToolStripMenuItem_Click() Sub
Dim Colour As New ColorDialog() Colour.Color = ColorTranslator.FromHtml(doc.bgColor) Colour.ShowDialog(Me) Try FormatBackground(Colour.Color) Catch ex As Exception ' Do nothing on Exception End Try
See Below:
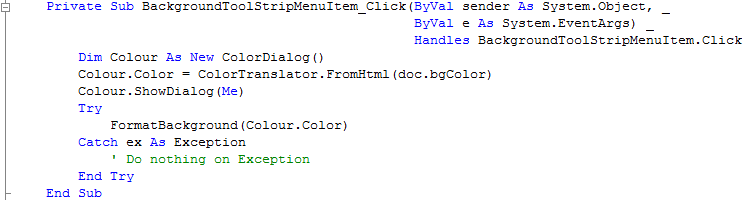
Step 32
Click on the [Design] Tab to view the form again, then Click on "Format" on the MenuStrip, then Double Click on the Menu Item Labeled "Align Left" (AlignLeftToolStripMenuItem) and type the following in the AlignLeftToolStripMenuItem_Click() Sub:
Command("JustifyLeft")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Format", then Double Click on the Menu Item Labeled "Align Centre" (AlignCentreToolStripMenuItem) and type the following in the AlignCentreToolStripMenuItem_Click() Sub:
Command("JustifyCenter")
Click on [Design] tab or double click on the frmMain entry in Solution Explorer again, click "Format", then Double Click on the Menu Item Labeled "Align Right" (AlignRightToolStripMenuItem) and type the following in the AlignRightToolStripMenuItem_Click() Sub:
Command("JustifyRight")
See Below:
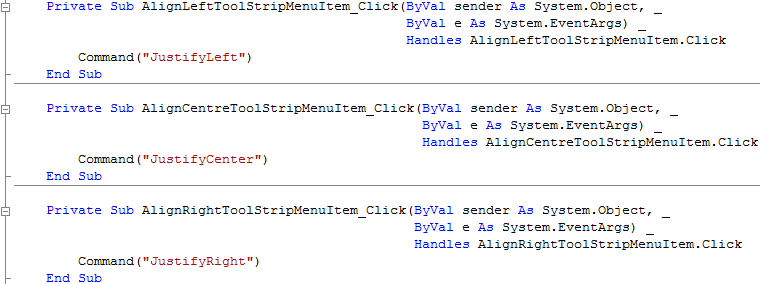
Step 33
Click on the [Design] Tab to view the form again, then Double Click on the Form (frmMain) and type the following in the frmMain_Load() Sub
webEditor.Navigate("about:blank") ' New Document doc = DirectCast(Me.webEditor.Document.DomDocument, _ mshtml.IHTMLDocument2) ' Prepare Document doc.designMode = "On" ' Design Mode Me.Text = "Web Editor - Untitled"
See Below:
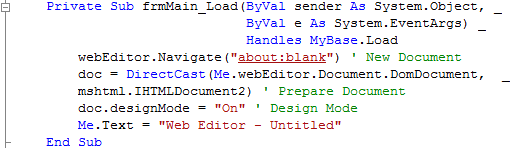
Step 34
Steps 34-37 are optional and just add Keyboard Shortcuts to the MenuItems, you don't have to do these if you don't want to!
Click on the [Design] Tab to view the form again, click on "New" on the MenuStrip then in the Properties box look for the "ShortcutKeys" option and
click on the Drop Down arrow where "None" appears. Check the "Ctrl" Checkbox in "Modifiers" and then in the "Key" dropdown list select "N",
see below:
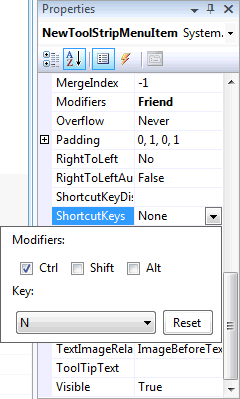
Step 35
Set the rest of the "File" MenuItem "ShortcutKeys" Properties except the "Exit" Menu Item, "Open" should be set to "Ctrl+O" and "Save" to "Ctrl+S", the File Menu should appear as below:
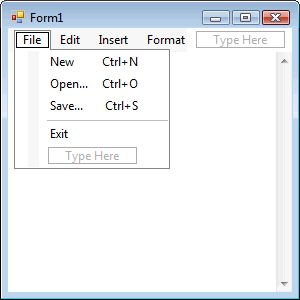
Step 36
Click on or select the "Edit" Menu and set the "ShortcutKeys" Properties, "Cut" should be set to "Ctrl+X", "Copy" to "Ctrl+C", "Paste" to "Ctrl+V", "Delete" should be just "Del" in the Keys drop-down list, "Select All" should be "Ctrl+A", "Time/Date" should be just "F5" from the Keys drop-down list. The Edit Menu should appear as below:
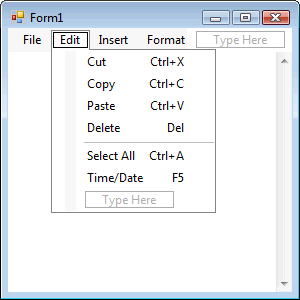
Step 37
Click on or select the "Format" Menu, select "Align Left" and in the "ShortcutKeys" property type "Ctrl+L", select "Align Centre" and type "Ctrl+E" in the "ShortcutKeys" property, "Align Right" should be set to "Ctrl+R". The Format Menu should appear as below:
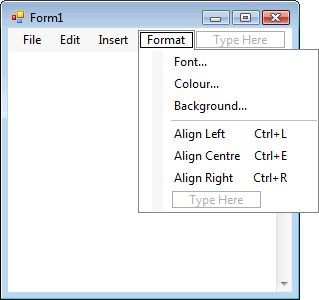
Step 38
Save the Project as you have now finished the application, then click on Start:

When you do the following will appear:
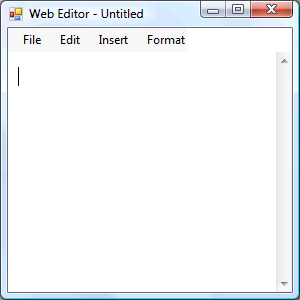
Step 39
You can start creating your own Web Page, or Open an existing one and edit it, see below:
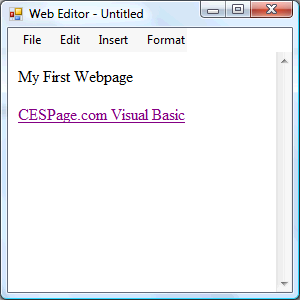
Step 40
Click File then Exit or click on the Close button
on the top right of Web Browser to end the application.
This program uses the mshtml component with the Web Browser control, see if you can add more functions using the Command function such as Bold, Italic and even Print, you can use this as the start of your very own Web Editor!